JSONCodec
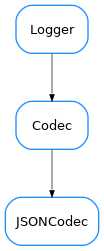
- class JSONCodec[source]
A codec able to encode/decode to/from json format. It uses the
json
module.Example:
>>> from taurus.core.util.codecs import CodecFactory >>> cf = CodecFactory() >>> codec = cf.getCodec('json') >>> >>> # first encode something >>> data = { 'hello' : 'world', 'goodbye' : 1000 } >>> format, encoded_data = codec.encode(("", data)) >>> print(encoded_data) '{"hello": "world", "goodbye": 1000}' >>> >>> # now decode it >>> format, decoded_data = codec.decode((format, encoded_data)) >>> print(decoded_data) {'hello': 'world', 'goodbye': 1000}
Import from
taurus.core.util.codecs
as:from taurus.core.util.codecs import JSONCodec